React, auth0, Gatsby, NextJS
Advanced React Security Patterns
91 Lessons
Security for React applications is a complex and wide-reaching topic. In this course, we'll look at implementing authentication and security best practices in React and many of the other tools that surround it. We'll explore various authentication methods, including JSON Web Tokens and cookies/sessions to determine the most suitable approach for various scenarios. The course covers essential security practices to safeguard data effectively and addresses common concerns about code inspection in browsers. Additionally, the course provides insights into applying authentication techniques in different React frameworks such as Gatsby and Next.js. It also delves into how to handle authentication in serverless functions, how to think about auth when it comes to GraphQL, and how to incorporate third-party authentication providers into our React apps.
Getting Started
1
Download the Code for the Course
2
Sign Up for MongoDB Atlas
3
Install Global Dependencies
4
Take a Tour of the Orbit App
5
Prerequisites for the Course
Refreshing JSON Web Tokens
6
Run the App and API
7
User Experience Problems with JWTs
8
How Refresh Tokens Work
9
Add an API Proxy
10
Add a Refresh Token Model
11
Save the Refresh Token in a Cookie
12
Add a Token Refresh Endpoint
13
Get a New Token in the Auth Debugger
14
Get a New Token on 401 Errors
15
Automatically Retry Post Requests
16
Add a Refresh Token Invalidation Endpoint
17
Add an Expiry Time to the Refresh Token
Switching to Cookies and Sessions
18
Add a CSRF Token
19
Run the App and API
20
Add an API Proxy
21
Install and Configure express-session
22
Set a Session on Login and Signup
23
Add a Session-Based Middleware
24
Add a Logout Endpoint
25
Add a Public Axios Instance
26
Create a User Info Endpoint
27
Check if the User is Authenticated
28
Refactor AuthContext
29
Refactor Login and Signup
30
Refactor the API
31
Add a Persistent Session Store
32
Strengthen the Session Cookie
Third Party Authentication Providers
33
Use the Auth0 Role in the React App
34
Request Scopes for an Access Token
35
Apply Scope Check Middleware to Endpoints
36
Add a Custom User ID with an Auth0 Rule
37
Allow Users to Log Out
38
Display the User's Name and Picture
39
Remove AuthContext, Login, and Signup
40
Renew Access Tokens
41
Create a User in Auth0
42
Set Up an API and Permissions
43
Add User Roles in Auth0
44
Use the Universal Login Screen
45
Install the Auth0-React SDK
46
Redirect Users to Auth0 to Log In
47
Use isLoading to Wait for Authentication
48
Use isAuthenticated to Check Auth Status
49
Get an Access Token from Auth0
50
Use a JWKS Verification Middleware
51
Augment the User's Profile with a Rule
52
Run the App and API
53
Why Use a Third-Party Auth Provider?
54
Sign Up for an Auth0 Account
55
Configure Application URLs
Authentication and Authorization for GraphQL
56
Run the App and API
57
Tour the GraphQL Implementation
58
Include a JWT in a GraphQL Request
59
Add the User to the GraphQL Context Object
60
Check Authorization in a Resolver
61
Add a Function to Check the User's Role
62
Define an Auth Schema Directive
63
Add a Custom Directive Class
64
Complete the Auth Directive Class
65
Apply the Auth Directive to the Schema
66
Use the User's Sub Claim
67
Redirect to the Login Page
Authentication and Authorization for GatsbyJS
68
Tour the Gatsby App Setup
69
Run the App and API
70
Wrap the Root Element with Providers
71
Create Client-Side Routes
72
Make Login and Signup be Client-Side Routes
73
Check the Environment when Building the App
Authentication and Authorization for Next.js
74
Install Dependencies and Run the App
75
Tour the Next.js Project Code
76
Make Calls for Data on the Server Side
77
Add an Authorization Middleware
78
Add an Admin Authorization Middleware
79
Check for Authentication on the Client
80
Check for the Admin Role on the Client
Serverless Authentication
81
Run the App and API
82
Sign Up for Netlify
83
Set Up a Directory for Serverless Functions
84
Create a Basic Serverless Function
85
Configure a Proxy to Netlify
86
Get Data from a Serverless Function
87
Check Authorization in a Serverless Function
88
Connect to a Database from a Serverless Function
89
Query a Database from a Serverless Function
90
Add a Role Check
91
Challenge: Complete the Remaining Endpoints
Getting Started
Refreshing JSON Web Tokens
Switching to Cookies and Sessions
Third Party Authentication Providers
Authentication and Authorization for GraphQL
Authentication and Authorization for GatsbyJS
Authentication and Authorization for Next.js
Serverless Authentication
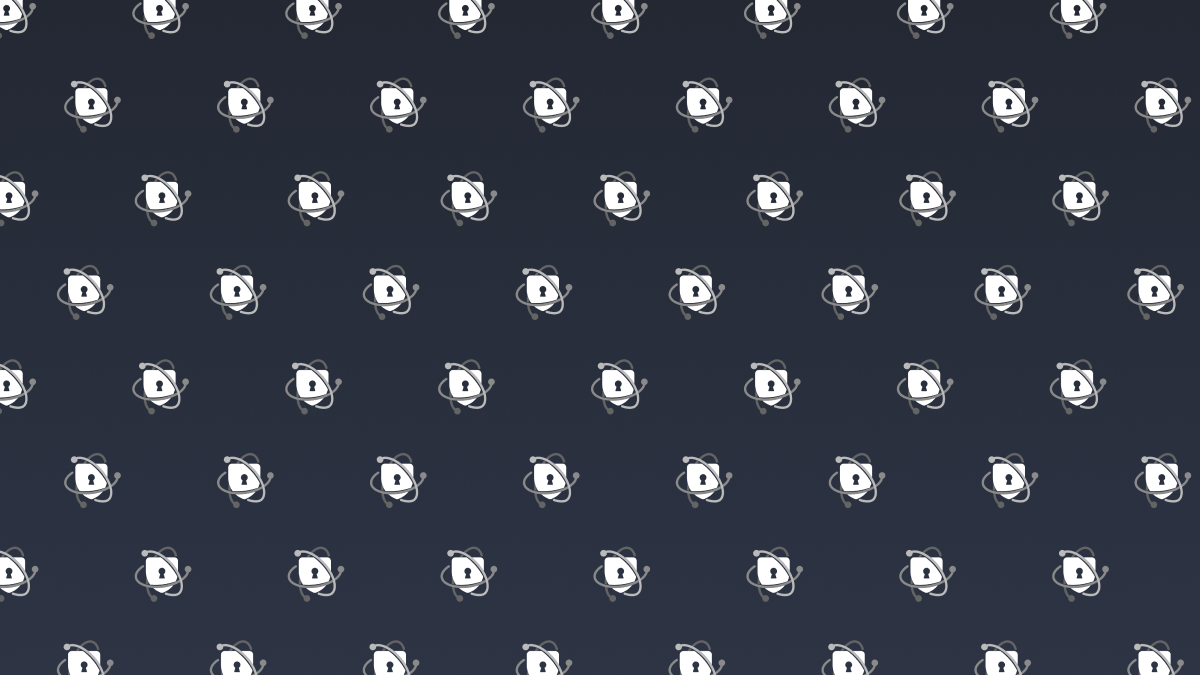