Learn modern full stack tech with real-world projects
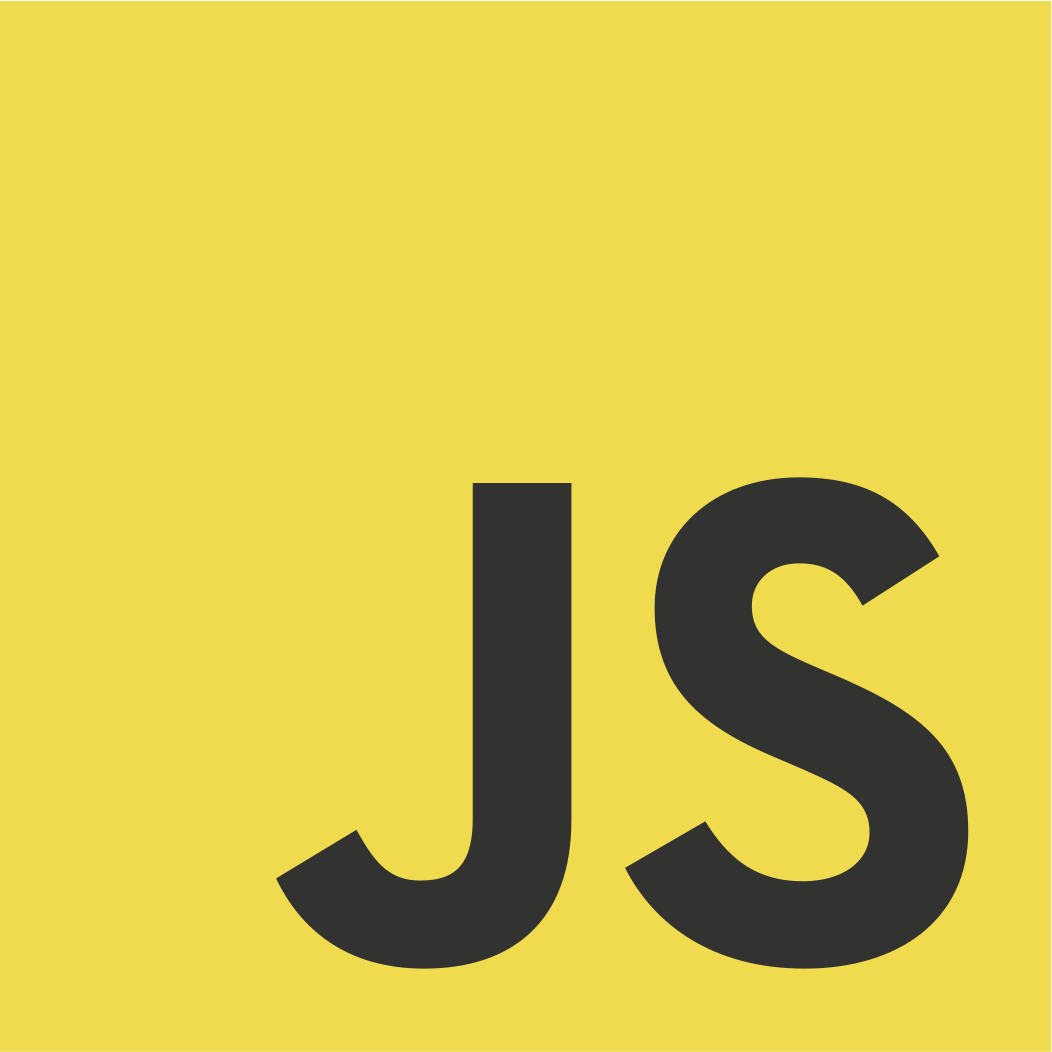
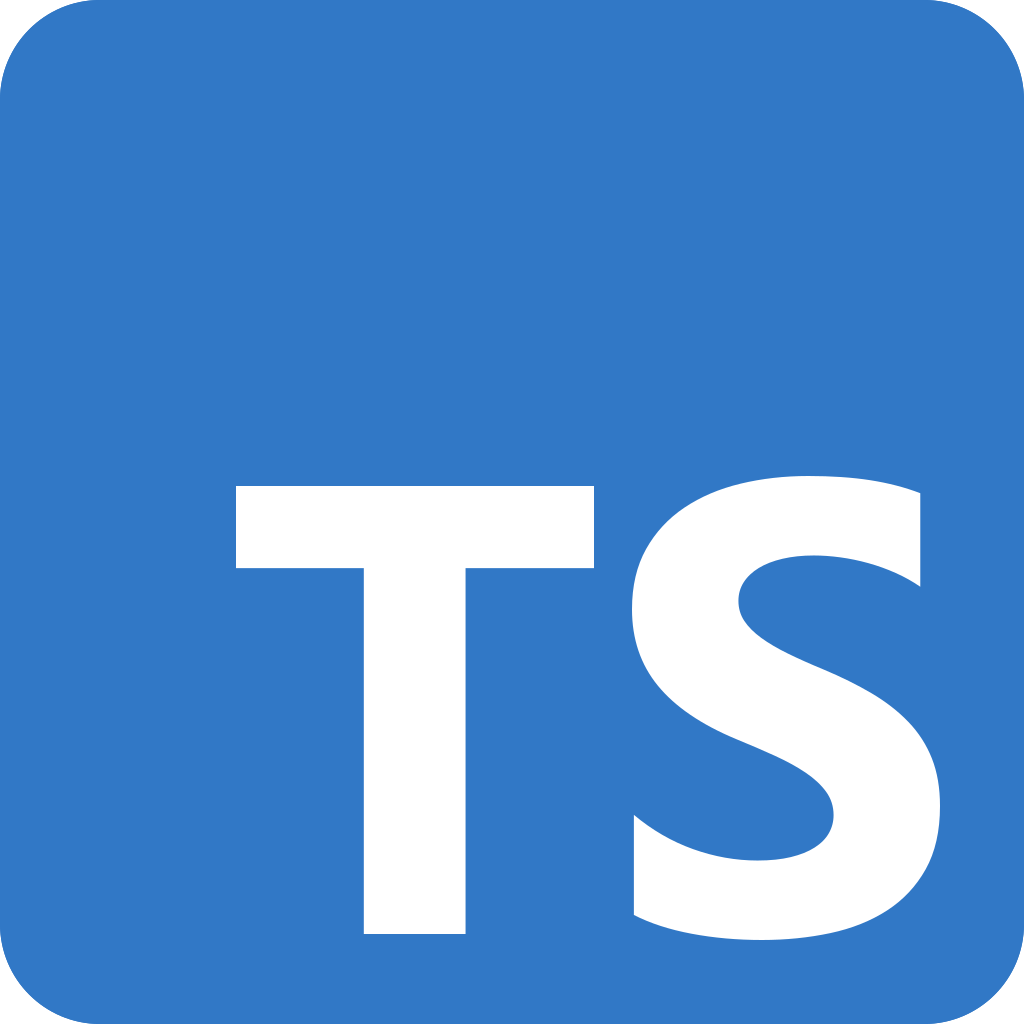
Getting Started with Prisma
23 Lessons
Welcome and Getting Started
1
Clone the Finished Project and Install Dependencies
2
Clone the Starting Point and Install Dependencies
3
Install the Prisma VS Code Extension
Installing and Initializing Prisma
4
Initialize Prisma in the Project
5
Install the Prisma CLI and Prisma Client
Our First Data Model
6
View and Manage Database Tables with Prisma Studio
7
Define a Data Model in the Prisma Schema
8
Add a Table to the Database with a Prisma Migration
Querying for Data from the App
9
Query for Data in getSeverSideProps
10
Enable Query Logging in Prisma Client
11
Create Data Using Prisma Client
Modelling Relations
12
Define Multiple Data Models in the Prisma Schema
13
Define a One-to-Many Relation in the Prisma Schema
14
Define a Many-to-Many Relation in the Prisma Schema
15
Create Related Records Using Prisma Studio
Prisma Queries for Full CRUD
16
Update and Delete Providers Data with Prisma Client
17
Update and Delete Posts Data with Prisma Client
18
Use Prisma Queries while Server-Side Rendering a Next.js App
19
Query for Data with Relations in getServerSideProps
20
Update and Delete Campaigns Data with Prisma Client
Wrapping Up
23
Use a Global Prisma Client Instance
Up and Running with Prisma and PlanetScale
11 Lessons
Prisma and PlanetScale
1
Create a Node and Prisma Backend
2
Create a Prisma Schema
3
Create Test Data Using Faker
4
Update the Schema and Open a Deploy Request
5
How to Handle Code Changes After Schema Updates
6
How to Handle Connection Strings
7
Switch from SQLite to PlanetScale
8
Install the PlanetScale CLI
9
What is PlanetScale?
10
Create a PlanetScale Database
11
Connect to a PlanetScale Database
React Security Fundamentals
39 Lessons
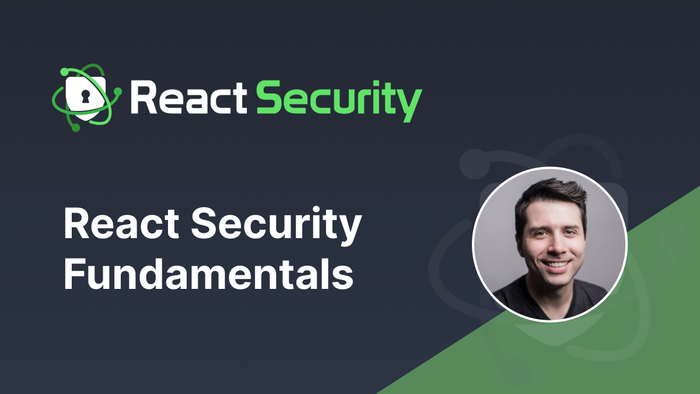
Getting Started
1
Tour the Application
2
Sign Up for MongoDB Atlas
3
Clone the Repo and Install Dependencies
4
Checkout the Start Branch
5
Run the React App and Express API
JSON Web Tokens
6
Anatomy of a JSON Web Token
7
Sign a JSON Web Token
8
JSON Web Token Dos and Don'ts
Signup and Login
9
View the Signup and Login Endpoints
10
Complete the User Signup Form
11
Complete the User Login Form
Handling Auth State
12
Navigate Conditionally Based on Auth State
13
Add Logout Functionality
14
Check the User's Role
15
Conditionally Display Sidebar Items
16
Set Auth State After Login
17
Use Auth State in UI Elements
18
Persist Auth State on Page Refresh
19
Check if the User is Currently Authenticated
Handling Client-Side Routing
20
Guard Client Side Routes Based on Auth State
21
Guard Client Side Routes Based on Role
Handling Authenticated HTTP Requests
22
Add a JWT to an Axios Request
23
Add an HTTP Interceptor to Axios
Protecting API Endpoints
24
Add a JWT Verification Middleware
25
Attach a User to the Request Object
26
Limit Access to Admin Users
27
Get the User ID from Requests
Advanced React Security Patterns
91 Lessons
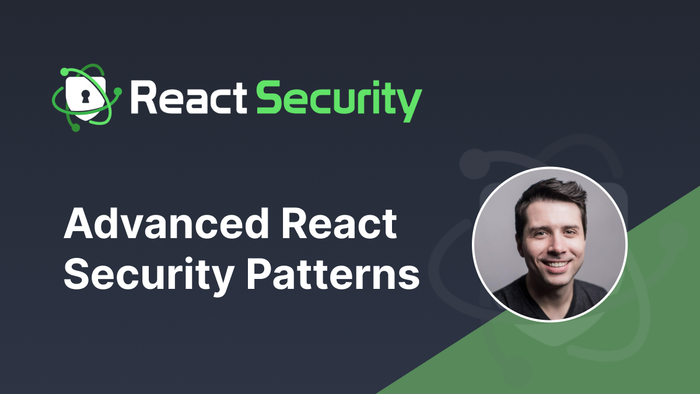
Getting Started
1
Download the Code for the Course
2
Sign Up for MongoDB Atlas
3
Install Global Dependencies
4
Take a Tour of the Orbit App
5
Prerequisites for the Course
Refreshing JSON Web Tokens
6
Run the App and API
7
User Experience Problems with JWTs
8
How Refresh Tokens Work
9
Add an API Proxy
10
Add a Refresh Token Model
11
Save the Refresh Token in a Cookie
12
Add a Token Refresh Endpoint
13
Get a New Token in the Auth Debugger
14
Get a New Token on 401 Errors
15
Automatically Retry Post Requests
16
Add a Refresh Token Invalidation Endpoint
17
Add an Expiry Time to the Refresh Token
Switching to Cookies and Sessions
18
Add a CSRF Token
19
Run the App and API
20
Add an API Proxy
21
Install and Configure express-session
22
Set a Session on Login and Signup
23
Add a Session-Based Middleware
24
Add a Logout Endpoint
25
Add a Public Axios Instance
26
Create a User Info Endpoint
27
Check if the User is Authenticated
28
Refactor AuthContext
29
Refactor Login and Signup
30
Refactor the API
31
Add a Persistent Session Store
32
Strengthen the Session Cookie
Third Party Authentication Providers
33
Use the Auth0 Role in the React App
34
Request Scopes for an Access Token
35
Apply Scope Check Middleware to Endpoints
36
Add a Custom User ID with an Auth0 Rule
37
Allow Users to Log Out
38
Display the User's Name and Picture
39
Remove AuthContext, Login, and Signup
40
Renew Access Tokens
41
Create a User in Auth0
42
Set Up an API and Permissions
43
Add User Roles in Auth0
44
Use the Universal Login Screen
45
Install the Auth0-React SDK
46
Redirect Users to Auth0 to Log In
47
Use isLoading to Wait for Authentication
48
Use isAuthenticated to Check Auth Status
49
Get an Access Token from Auth0
50
Use a JWKS Verification Middleware
51
Augment the User's Profile with a Rule
52
Run the App and API
53
Why Use a Third-Party Auth Provider?
54
Sign Up for an Auth0 Account
55
Configure Application URLs
Authentication and Authorization for GraphQL
56
Run the App and API
57
Tour the GraphQL Implementation
58
Include a JWT in a GraphQL Request
59
Add the User to the GraphQL Context Object
60
Check Authorization in a Resolver
61
Add a Function to Check the User's Role
62
Define an Auth Schema Directive
63
Add a Custom Directive Class
64
Complete the Auth Directive Class
65
Apply the Auth Directive to the Schema
66
Use the User's Sub Claim
67
Redirect to the Login Page
Authentication and Authorization for GatsbyJS
68
Tour the Gatsby App Setup
69
Run the App and API
70
Wrap the Root Element with Providers
71
Create Client-Side Routes
72
Make Login and Signup be Client-Side Routes
73
Check the Environment when Building the App
Authentication and Authorization for Next.js
74
Install Dependencies and Run the App
75
Tour the Next.js Project Code
76
Make Calls for Data on the Server Side
77
Add an Authorization Middleware
78
Add an Admin Authorization Middleware
79
Check for Authentication on the Client
80
Check for the Admin Role on the Client
Serverless Authentication
81
Run the App and API
82
Sign Up for Netlify
83
Set Up a Directory for Serverless Functions
84
Create a Basic Serverless Function
85
Configure a Proxy to Netlify
86
Get Data from a Serverless Function
87
Check Authorization in a Serverless Function
88
Connect to a Database from a Serverless Function
89
Query a Database from a Serverless Function
90
Add a Role Check
91
Challenge: Complete the Remaining Endpoints
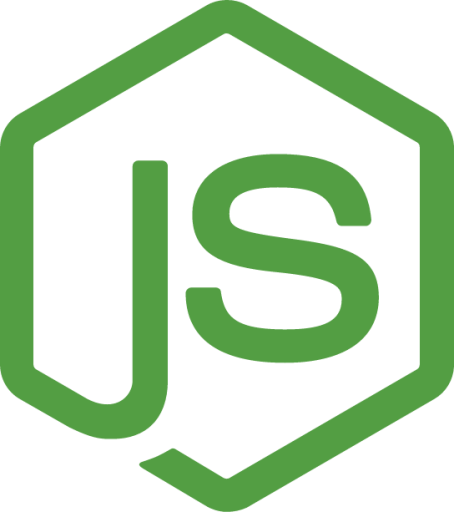
What's New in Node 22?
6 Lessons
Prisma vs Drizzle: Comparing the Top Type-Safe ORMs
14 Lessons
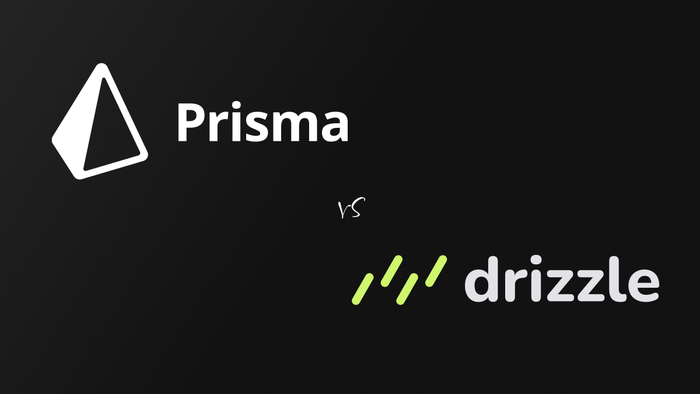
Prisma vs Drizzle
1
Install Dependencies and Set Up Initial Files
2
Create the Prisma and Drizzle Databases
3
Seed the Prisma Database
4
Seed the Drizzle Database
5
Run a Simple Find Query with Prisma
6
Run a Simple Find Query with Drizzle
7
Handling Relations with Prisma
8
Handling Relations with Drizzle
9
One to Many Relations with Drizzle
10
Many to Many Relations with Drizzle
11
All Prisma CRUD Operators
12
All Drizzle CRUD Operators
13
Using Prisma and Drizzle Studio
14
Using Prisma and Drizzle Migrate