React
React Security Fundamentals
39 Lessons
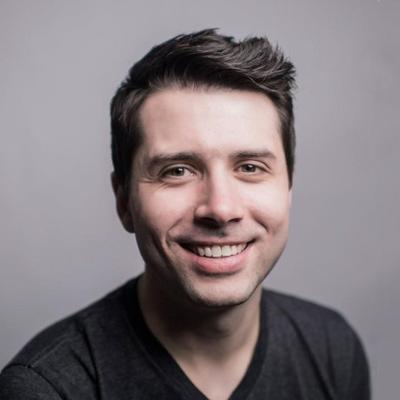
Ryan Chenkie
Full Stack Instructor
Application security is often thought of as something that is mostly a backend concern. In reality, there are a ton of important security considerations that need to be thought about when it comes to the front end as well and React is no exception. In this course, we explore some of the biggest security threats that exist for React applications and how we can mitigate them. Using sample application called Orbit, we'll explore topics like JSON Web Tokens, how to implement a secure login/signup form, how to implement role-based authorization, and much more.
Getting Started
1
Tour the Application
2
Checkout the Start Branch
3
Run the React App and Express API
4
Sign Up for MongoDB Atlas
5
Clone the Repo and Install Dependencies
JSON Web Tokens
6
Anatomy of a JSON Web Token
7
Sign a JSON Web Token
8
JSON Web Token Dos and Don'ts
Signup and Login
9
View the Signup and Login Endpoints
10
Complete the User Signup Form
11
Complete the User Login Form
Handling Auth State
12
Add Logout Functionality
13
Check the User's Role
14
Conditionally Display Sidebar Items
15
Set Auth State After Login
16
Use Auth State in UI Elements
17
Check if the User is Currently Authenticated
18
Persist Auth State on Page Refresh
19
Navigate Conditionally Based on Auth State
Handling Client-Side Routing
20
Guard Client Side Routes Based on Auth State
21
Guard Client Side Routes Based on Role
Handling Authenticated HTTP Requests
22
Add a JWT to an Axios Request
23
Add an HTTP Interceptor to Axios
Protecting API Endpoints
24
Add a JWT Verification Middleware
25
Attach a User to the Request Object
26
Limit Access to Admin Users
27
Get the User ID from Requests
Getting Started
JSON Web Tokens
Signup and Login
Handling Auth State
Handling Client-Side Routing
Handling Authenticated HTTP Requests
Protecting API Endpoints
Hardening the Application
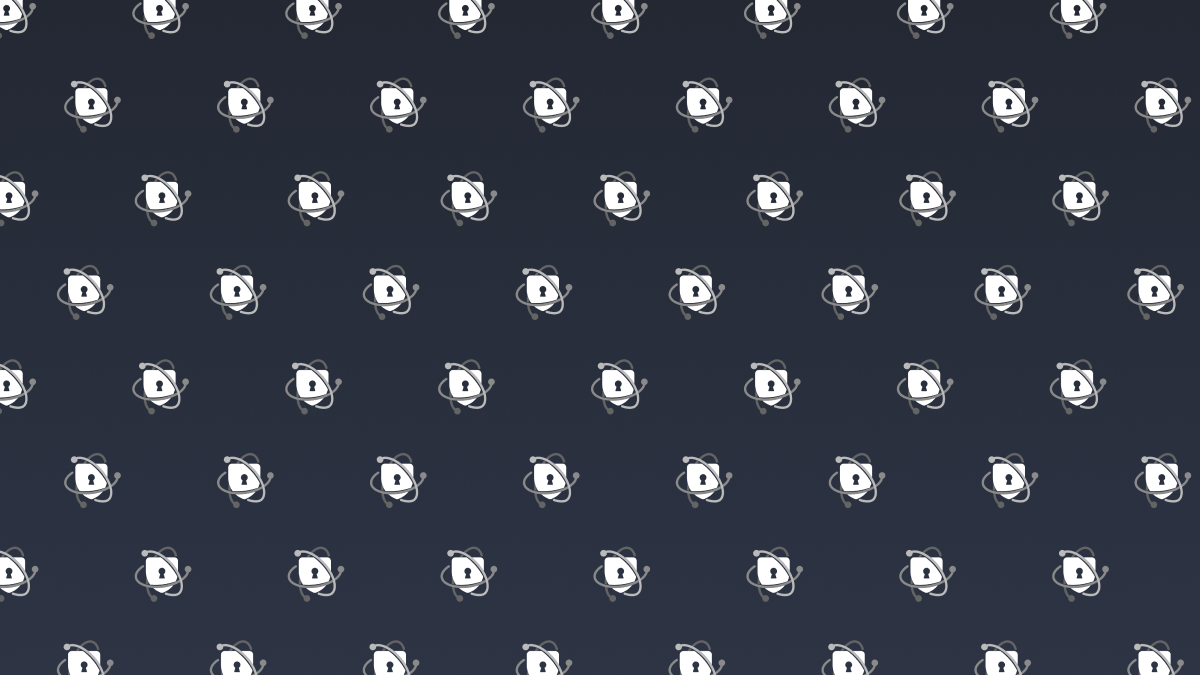